Table of Contents
ToggleIntroduction
In today’s dynamic software development landscape, microservices architecture has become a popular approach for building scalable and resilient applications. One of the key components in ensuring the smooth operation of a microservices-based system is service discovery. This article delves into what it is, why it’s essential, and how it integrates with microservices architecture to enhance performance and reliability.
What Is Service Discovery?
Service discovery is the process by which services in a microservices architecture automatically locate and communicate with each other. Unlike monolithic applications where service endpoints are often hardcoded, it allows microservices to dynamically find and interact with each other.
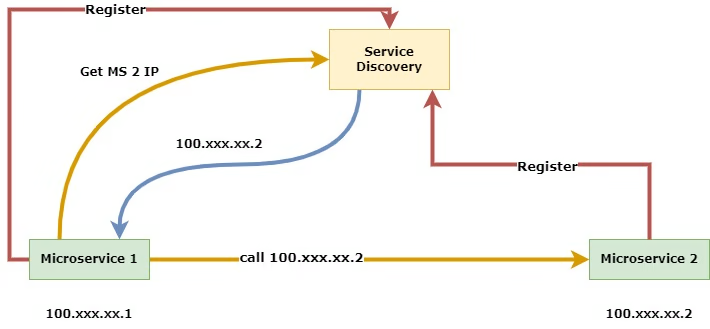
Why Service Discovery Is Crucial
- Dynamic Scaling: Microservices often scale up or down based on demand. Service discovery ensures that new instances are registered and old ones are deregistered without manual intervention.
- Fault Tolerance: In case of a service failure, it automatically reroute requests to healthy instances, enhancing system resilience.
- Load Balancing: It helps distribute incoming requests across multiple service instances, optimizing resource usage and improving performance.
Types of Service Discovery
Client-Side Service Discovery
In client-side service discovery, the client is responsible for querying the service registry to find the location of a service instance. The client then makes a direct request to the discovered service.
Example: Suppose you have a microservice that handles user authentication. When another service needs to authenticate a user, it queries the service registry for the authentication service’s address and communicates directly with it.
Code Example:
// Example using Netflix Eureka in a Spring Boot application
@RestController
public class AuthController {
@Autowired
private RestTemplate restTemplate;
@GetMapping("/login")
public ResponseEntity<String> loginUser(@RequestParam String username, @RequestParam String password) {
// Query the service registry for the auth service instance
String authServiceUrl = "http://auth-service/login";
// Directly call the auth service
ResponseEntity<String> response = restTemplate.postForEntity(authServiceUrl, new LoginRequest(username, password), String.class);
return response;
}
}
Server-Side Service Discovery
Server-side service discovery involves a load balancer or API gateway that handles the lookup and routing of requests to the appropriate service instance.
Example: In this approach, a load balancer like Nginx or HAProxy sits in front of your services. When a request comes in, the load balancer queries the service registry and forwards the request to a suitable service instance.
Code Example:
# Example Nginx configuration for load balancing
http {
upstream auth_service {
server auth-service-instance-1:8080;
server auth-service-instance-2:8080;
}
server {
listen 80;
location /login {
proxy_pass http://auth_service;
}
}
}
Hybrid Service Discovery
Hybrid service discovery combines client-side and server-side approaches, leveraging the benefits of both methods. Clients may perform initial service lookups and then use a server-side load balancer for routing.
How Service Discovery Enhances Microservices
Dynamic Scaling
In a microservices environment, services might be added or removed frequently. Service discovery allows the system to adapt to these changes automatically.
Example: During a flash sale, your e-commerce application might spin up additional instances of the checkout service. Service discovery ensures that these new instances are immediately available to handle user requests.
Fault Tolerance
Service discovery enhances fault tolerance by continuously updating the service registry with the status of service instances. If an instance fails, it is removed from the registry, and requests are redirected to other healthy instances.
Example: If a user service instance crashes, service discovery will update the registry to exclude this instance, and the system will route requests to other available instances.
Load Balancing
Load balancing distributes incoming traffic evenly across multiple instances of a service, preventing any single instance from becoming overwhelmed.
Example: A payment service might have multiple instances running behind a load balancer. Service discovery helps ensure that traffic is distributed evenly across these instances.
Service Discovery Mechanisms
DNS-Based Service Discovery
DNS-based service discovery uses DNS records to manage service locations. This method is simple and relies on DNS to resolve service names to IP addresses.
Example: You might use DNS records to map the service name auth-service to the IP addresses of its instances.
API Gateway-Based Service Discovery
API gateways can perform service discovery by routing requests based on the current registry data. Popular API gateways like Kong and AWS API Gateway support service discovery functionalities.
Example: An API gateway routes incoming requests to the appropriate service instance based on the service registry data.
Service Registry and Discovery Tools
Several tools facilitate service discovery, offering features like health checks and automatic registration. Popular options include:
- Consul: Provides service registration, health checks, and a powerful DNS interface.
- Eureka: A REST-based service registry with client-side load balancing.
- Zookeeper: A distributed coordination service that supports service discovery.
Implementing Service Discovery
Choosing the Right Service Discovery Mechanism
Selecting the appropriate service discovery mechanism depends on your system’s requirements, including scalability, complexity, and existing infrastructure.
Integration with Existing Systems
Integrate service discovery tools with your current system setup, ensuring minimal disruption and maximum benefit.
Best Practices for Implementation
- Consistency: Maintain consistent registration and discovery practices.
- Health Checks: Regularly perform health checks to ensure the registry contains only healthy instances.
- Documentation: Document your service discovery setup to facilitate troubleshooting and maintenance.
Common Challenges in Service Discovery
Managing Service Registries
Keeping service registries up-to-date in dynamic environments can be challenging. Ensure that service instances are correctly registered and deregistered as needed.
Handling Service Failures
Service discovery must effectively handle service failures by updating the registry to exclude failed instances promptly.
Ensuring Security
Protect your infrastructure from unauthorized access and potential attacks. Implement security measures to safeguard service registration and discovery processes.
Addressing Latency Issues
Optimize service discovery mechanisms to minimize latency and ensure fast, reliable service lookups.
Best Practices for Effective Service Discovery
Regular Updates and Maintenance
Keep your service discovery infrastructure updated and maintained to handle changes and new requirements effectively.
Monitoring and Analytics
Implement monitoring and analytics to track the performance of your setup and identify areas for improvement.
Security Measures
Apply robust security measures to protect your infrastructure from potential threats.
Testing and Validation
Regularly test and validate your setup to ensure it operates correctly and meets your requirements.
The Future of Service Discovery
Emerging Trends and Technologies
Stay informed about emerging trends and technologies that could have impact, such as advancements in cloud computing and container orchestration.
Impact of Advances in Cloud Computing
Reflect on how innovations in cloud computing will impact the future of service discovery and redefine the methods for managing and locating services.
Predictions for Service Discovery Evolution
Explore predictions for how service discovery will evolve and what new developments to expect in the coming years.
Conclusion
Service discovery is a critical aspect of microservices architecture, facilitating efficient communication between services and enhancing overall system performance. By understanding its role and implementing best practices, you can ensure that your microservices architecture remains robust, scalable, and reliable.
FAQs
What is the difference between client-side and server-side service discovery?
Client-side discovery involves the client querying the service registry directly, while server-side discovery uses a load balancer or API gateway to manage service lookups.
How does service discovery impact load balancing?
it help distribute requests across multiple service instances, improving load balancing and ensuring even traffic distribution.
Can service discovery handle high traffic volumes?
Yes, It is designed to handle high traffic volumes by efficiently routing requests and scaling service instances as needed.
What tools are available for service discovery?
Popular tools include Consul, Eureka, and Zookeeper, each offering various features and capabilities for managing service locations.
How can service discovery improve fault tolerance?
It improve fault tolerance by automatically updating the service registry to exclude failed instances, preventing clients from attempting to connect to unavailable services.
One Response