Table of Contents
ToggleIntroduction
In the modern era of web development, real-time updates are crucial for creating interactive and dynamic user experiences. Whether it’s live sports scores, stock market updates, or notifications in social media applications, users expect immediate data without constantly refreshing their pages. Server-Sent Events (SSE) in Java provide a straightforward way to implement such real-time capabilities in your applications.
This article will delve into the Server-Sent Events concept, their Java implementation, and how they can be used to deliver a seamless real-time experience. We’ll also provide code examples to help you understand and apply this technology effectively.
What Are Server-Sent Events (SSE)?
Server-Sent Events (SSE) is a technology that enables servers to push updates to clients over a single, long-lived HTTP connection. Unlike WebSockets, which support bidirectional communication, SSE is unidirectional, meaning the server can only send data to the client. This makes SSE ideal for use cases where real-time data, such as live feeds, notifications, or updates, must be streamed from the server to the client.
Key Characteristics of SSE
- Unidirectional Data Flow: Data flows from the server to the client.
- Uses HTTP/1.1: SSE operates over the standard HTTP protocol.
- Automatic Reconnection: The client automatically reconnects if the connection is lost.
- Simple and Lightweight: SSE is easier to implement and more lightweight compared to WebSockets.
How Do Server-Sent Events Work?
SSE establishes a persistent HTTP connection between the client and the server. Here’s a brief overview of how this communication happens:
- Client Request: The client makes an HTTP request to the server to initiate an SSE connection.
- Server Response: The server responds by keeping the connection open and sending updates as they become available.
- Client Receives Updates: The client listens for updates and processes them in real time.
- Automatic Reconnection: If the connection drops, the client attempts to reconnect automatically.
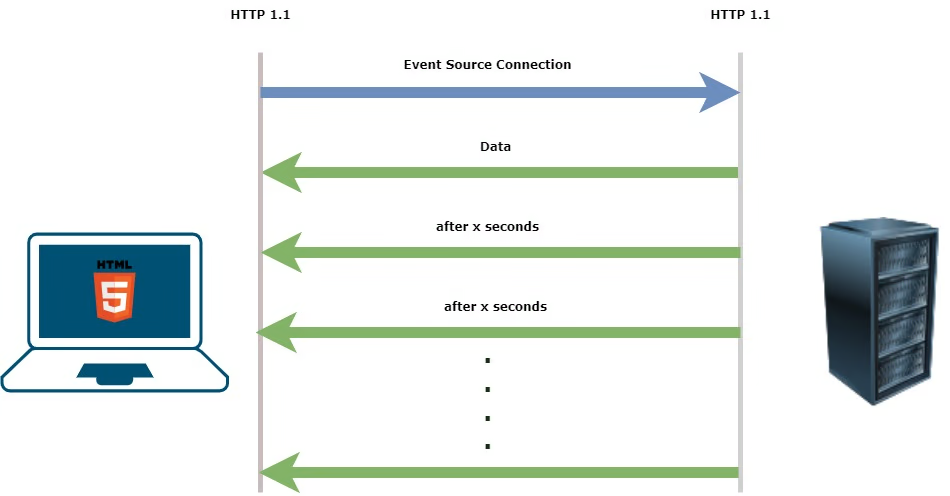
Implementing Server-Sent Events in Java
Java provides robust support for implementing SSE through its various libraries and frameworks. In this section, we’ll walk through the implementation of SSE using Java with the Spring Boot framework.
Step 1: Setting Up the Spring Boot Application
First, create a new Spring Boot project. You can do this using Spring Initializr or by setting up the project manually. Make sure to include the necessary dependencies, such as spring-boot-starter-web. To learn more about Spring boot-related topics, please read my Spring related blog page
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
Step 2: Creating the SSE Endpoint
Next, create a controller that will handle the SSE connection and stream data to the client. The controller will use Spring’s SseEmitter class to manage the SSE connection.
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RestController;
import org.springframework.web.servlet.mvc.method.annotation.SseEmitter;
import java.io.IOException;
import java.util.concurrent.Executors;
import java.util.concurrent.TimeUnit;
@RestController
public class SseController {
@GetMapping("/stream-sse")
public SseEmitter streamSse() {
SseEmitter emitter = new SseEmitter();
Executors.newSingleThreadScheduledExecutor().scheduleAtFixedRate(() -> {
try {
emitter.send(SseEmitter.event().data("Real-time update: " + System.currentTimeMillis()));
} catch (IOException e) {
emitter.completeWithError(e);
}
}, 0, 5, TimeUnit.SECONDS);
return emitter;
}
}
In this example, the /stream-sse endpoint initiates an SSE connection with the client. The server sends updates every 5 seconds with the current timestamp. The SseEmitter class handles the SSE connection, including sending data and dealing with errors.
Step 3: Setting Up the Client-Side Code
Now, let’s create the client-side code that will connect to the SSE endpoint and display the real-time updates.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Java Server-Sent Events Example</title>
</head>
<body>
<h1>Real-Time Updates</h1>
<div id="updates"></div>
<script>
const eventSource = new EventSource('/stream-sse');
eventSource.onmessage = function(event) {
const updatesDiv = document.getElementById('updates');
const newElement = document.createElement('p');
newElement.textContent = event.data;
updatesDiv.appendChild(newElement);
};
eventSource.onerror = function() {
console.error('SSE connection failed. Attempting to reconnect...');
};
</script>
</body>
</html>
The client-side code uses the EventSource API to connect to the /stream-sse endpoint. It listens for incoming messages and displays them on the web page. The onerror event handler is used to log any connection issues and attempt reconnection.
Advantages of Using Server-Sent Events in Java
Using SSE in Java comes with several advantages:
- Ease of Implementation: SSE is easier to implement than WebSockets, especially in scenarios where only unidirectional data flow is required.
- Automatic Reconnection: Java’s SseEmitter and the client’s EventSource API handle automatic reconnection, making the process seamless for users.
- Scalability: SSE operates over standard HTTP, making it easier to scale using traditional load balancers and proxies.
- Efficiency: SSE is more efficient than polling because it only sends data when there are updates, reducing unnecessary network traffic.
Use Cases for Server-Sent Events in Java
SSE is well-suited for a variety of use cases, including:
- Real-Time Notifications: Send real-time alerts and notifications to users.
- Live Data Feeds: Stream live data, such as sports scores or stock prices, to clients.
- Chat Applications: Implement simple chat applications where messages are pushed from the server to the client.
- Monitoring Dashboards: Provide real-time updates for monitoring tools and dashboards.
Limitations of Server-Sent Events
While SSE is a powerful tool, it does have some limitations:
- Unidirectional: SSE only supports server-to-client communication. For bidirectional communication, consider using WebSockets.
- Limited Browser Support: While SSE is supported by most modern browsers, it may not work in older browsers. Always check compatibility before using SSE in production.
- Connection Limits: Some environments, like certain load balancers or proxies, may limit the number of open connections, which can impact the scalability of SSE.
Conclusion
Server-Sent Events offer a simple yet effective way to implement real-time communication in your Java applications. By leveraging Java’s robust support for SSE, you can create dynamic and responsive applications that provide users with real-time updates.
Whether you’re building a notification system, a live data feed, or a monitoring dashboard, SSE can help you deliver a seamless real-time experience. With the code examples provided, you should now have a solid foundation to start implementing SSE in your own Java projects.
FAQs
1. What are Server-Sent Events (SSE) in Java?
Server-Sent Events (SSE) in Java is a technology that allows servers to push real-time updates to clients over a single, long-lived HTTP connection. SSE is unidirectional, meaning that the server sends data to the client, but the client does not send data back to the server over the same connection.
2. How do Server-Sent Events differ from WebSockets?
While both SSE and WebSockets enable real-time communication, they differ in their approach. SSE is unidirectional, sending data only from the server to the client, and operates over standard HTTP. WebSockets, on the other hand, support bidirectional communication, allowing data to flow both ways between the server and the client, and require a dedicated WebSocket protocol.
3. What are the advantages of using SSE over other real-time communication methods?
SSE is simpler to implement than WebSockets, particularly for use cases where only server-to-client communication is needed. It also supports automatic reconnection, uses standard HTTP, and is lightweight, making it efficient for many real-time applications.
4. What are some common use cases for Server-Sent Events in Java?
Common use cases for SSE in Java include real-time notifications, live data feeds (e.g., sports scores or stock prices), simple chat applications, and monitoring dashboards. SSE is ideal for scenarios where the server needs to push updates to the client as soon as they occur.
5. Are there any limitations to using Server-Sent Events in Java?
Yes, there are some limitations to using SSE. It only supports unidirectional communication (server to client), may not be supported by all browsers (especially older ones), and can encounter connection limits in certain environments, which may impact scalability.