Table of Contents
ToggleDefine Maven
What is Maven
Maven is a Java-based automation tool primarily used for Java projects. Not only Java, but Maven is also capable of managing and building code projects written in C#, Ruby, Scala, and other programming languages. It eases the process of project build, handling dependencies, and project documentation in a simple manner. Before Maven, different projects used to have their own Ant build files, and the dependency jars were used to check in the project code repositories. Maven is the best build tool for Spring Boot projects and for developing Microservices applications

The Purpose of Using Maven
Maven’s primary goal is to allow a developer to comprehend the complete state of a development effort in the shortest period. Using Maven, you can manage your project lifecycle, dependencies, and contributions from multiple team members effectively. This tool helps to ensure that everyone on your team is using the same versions of libraries and plugins, leading to a smoother collaboration.
Key Features of Maven

- Ease of setup: Simple project setup that follows best practices – get a new project or module started in seconds
- Consistency: Consistent usage across all projects – means no ramp-up time for new developers coming onto a project
- Model-based builds: Maven is able to build any number of projects into predefined output types such as a JAR, WAR, or distribution based on metadata about the project, without the need to do any scripting in most cases.
- Dependency Management: Automatically downloads and manages libraries needed for your project, reducing conflicts and streamlining builds.
- Project Lifecycle Management: Helps in organizing the entire build lifecycle from compilation to packaging to deployment in a structured way.
- Integration with IDEs: Works well with popular Integrated Development Environments (IDEs) like Eclipse and IntelliJ.
Setting Up Maven
Installing Maven on Your System
One minor drawback of Java I would say is that it requires installation on your machine so you would be required to download and install it in your system. The latest version can be downloaded from Apache Maven official website. The installation process is pretty straightforward:
- Download the binary zip file.
- Extract the zip file to a preferred directory on your device.
As a personal tip, I like to keep my development tools organized in a folder called “DevTools” to easily find what I need.
Configuring Environment Variables
After installation, you need to configure your environment:
- Set the MAVEN_HOME variable: Point this variable to the directory where you extracted Maven.
- Update the PATH variable: Add Maven’s bin directory to your system PATH. This allows you to run Maven commands from your command line.
Creating Your First Maven Project
Creation of a maven project just required any of your system’s command line tool. Open the command line tool and run below command:
mvn archetype:generate -DgroupId=com.example -DartifactId=my-first-app -DarchetypeArtifactId= maven-archetype-quickstart -DinteractiveMode=false
This command sets up a basic project structure. Trust me, seeing your project files generated automatically is quite satisfying!
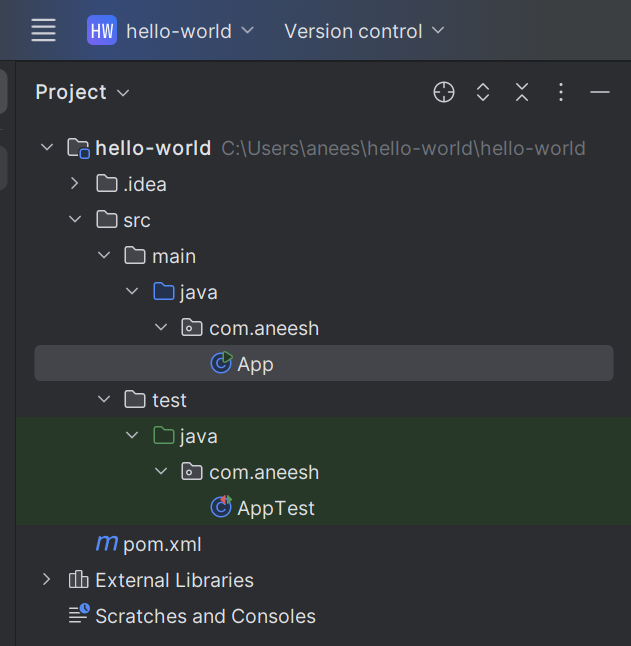
Since Maven follows the convention about configuration. Therefore, it defines some default settings (which can be overridden):
- Source code files should be in src/main/java
- Test files should be located in src/test/java
- pom.xml should be located in the root directory
Understanding Maven’s Project Object Model (POM)
Structure of the POM File
The Project Object Model (POM) file is an XML file named pom.xml. It contains information about the project and is the core of Maven’s configuration.
Here’s a simple breakdown of its structure:
- Project identifiers (groupId, artifactId, version)
- Dependencies
- Build settings
Key Elements in the POM
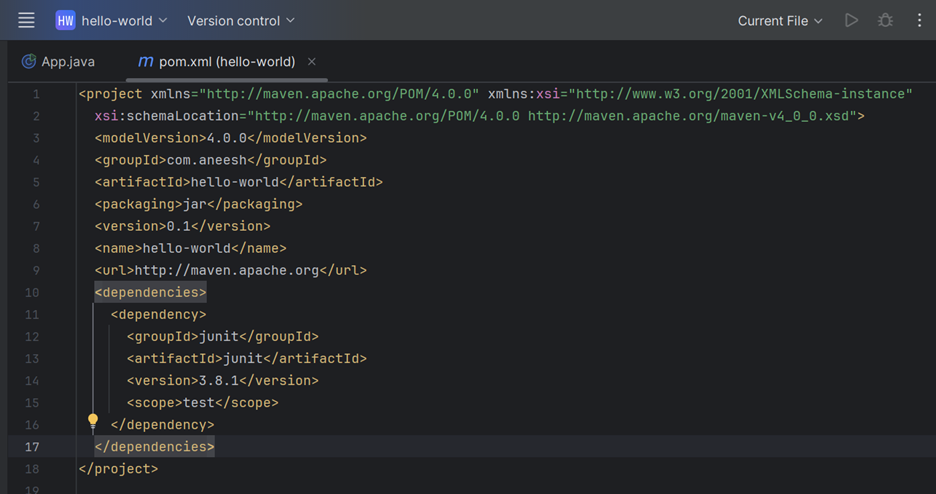
Some essential elements within the POM file include:
- groupId: Defines the group or organization that the project belongs to.
- artifactId: A unique identifier for the project.
- version: The version of the project.
- dependencies: Lists the external libraries your project relies on.
Managing Dependencies with the POM
Managing dependencies in Maven is incredibly user-friendly. To add a dependency, simply insert it into your POM file under the <dependencies> section. For instance:
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>3.8.1</version>
<scope>test</scope>
</dependency>
This practice not only saves you time but also minimizes the risk of version conflicts among libraries.
Building and Managing Projects with Maven
Lifecycle Phases of a Maven Build
The Maven build lifecycle consists of a series of phases that are executed when a Maven build is run. There are three build lifecycles: standard, clean, and site. The standard lifecycle includes the following phases:
- validate: Checks that project is valid and all necessary information is provided
- compile: Compiles the source code( including test)
- test: Tests the unit test case classes present in src/test folder
- package: Packages the compiled source code to the desired package type(i.e. jar/war etc.)
- verify: Checking the results of integration tests to ensure that the quality criteria are met
- install: Installs the built package to the local repository
- deploy: Copies the built package to the remote repository
The above phases run in sequential order to complete the standard life cycle. To understand it better let’s run these commands
mvn validate
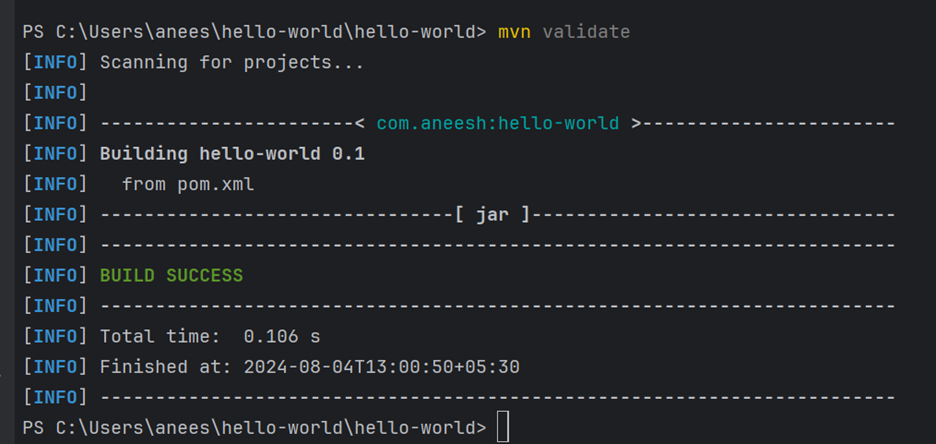
mvn clean: Removes the target directory where compiled files reside.

mvn compile: Once compiled, src files can be seen in ‘target’ folder

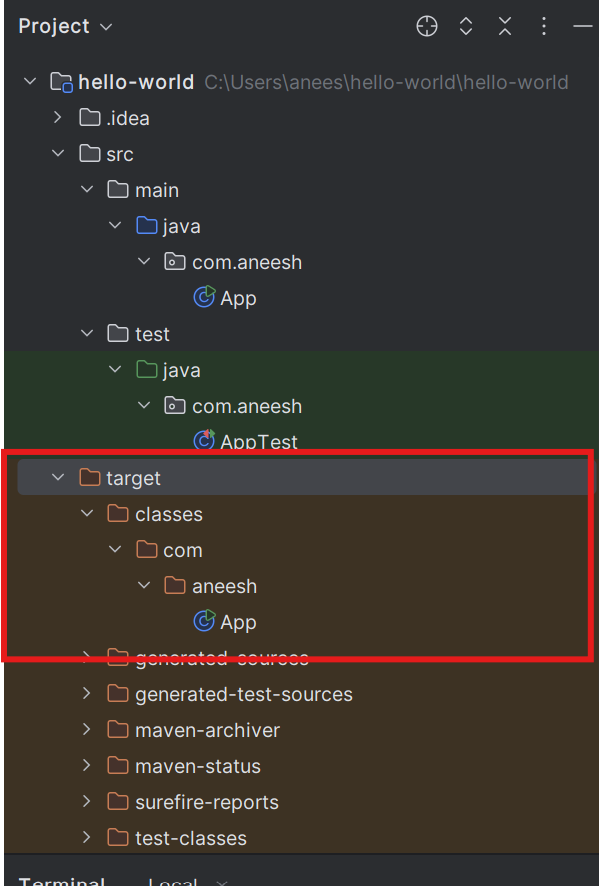
mvn test-compile: compiles the test classes. Again, test classes will be compiled into the target folder
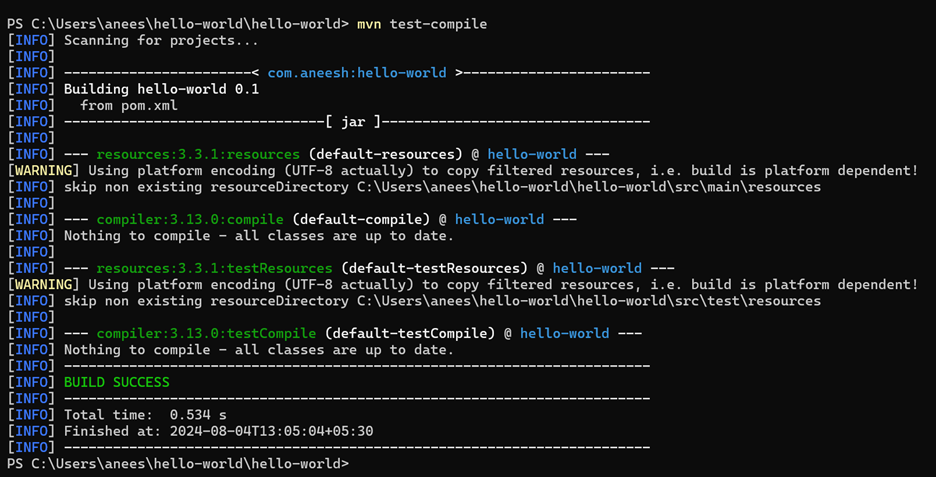
mvn verify: It compiles src and test files, runs the test, and verifies that quality criteria are met.
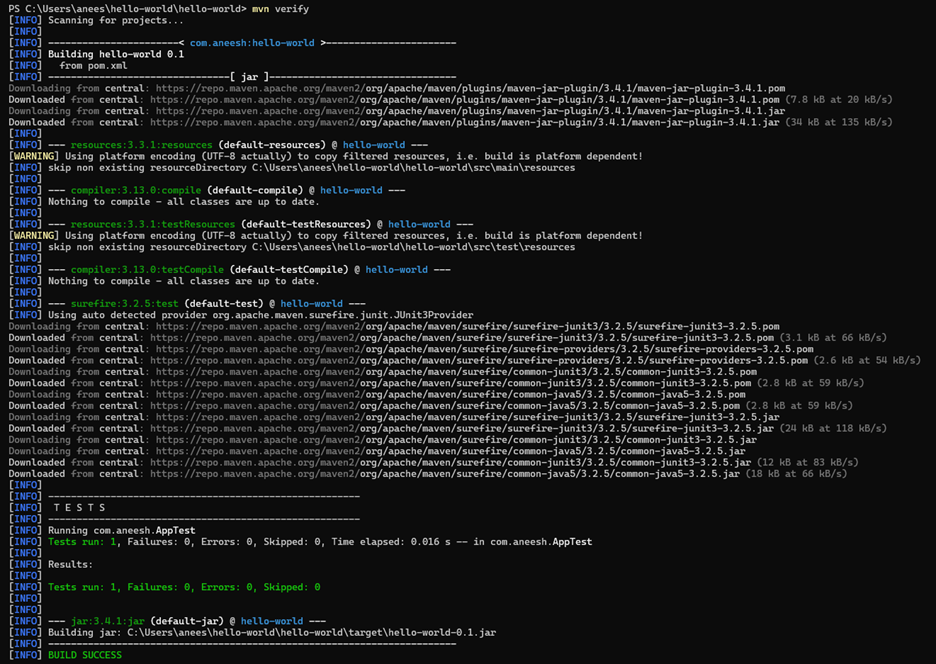
mvn install: In the installation phase, Maven first validates, compiles all source code files, packages the artifacts as per pom.xml, verifies the package, and then installs it in the local repository(.m2/repository). This installed package can be used as a dependency in other projects and hence eases the dependency between the different projects

Effective Versioning and Dependency Management
Having a clear versioning strategy is crucial. Utilizing Maven allows you to specify version ranges for dependencies that keep your project updated without breaking changes. This way, you can focus on building new features rather than wrestling with library updates.
Advanced Maven Concepts
Maven Repositories: Local and Remote
Maven manages libraries using repositories. These are:
- Local Repository: A specific folder on your machine (usually
~/.m2/repository
) that stores downloaded libraries. - Remote Repository: Online repositories like Maven Central where libraries are fetched if not already available locally.
When a Maven project is built, it looks for the dependency in the local repository and if that is not found, then it downloads the dependency from the remote repository and saves it to the local repository. This avoids the downloading of dependencies each time the code is built.
Utilizing Maven Plugins
Maven can be extended with plugins, adding functionality to various phases of your project’s lifecycle. Plugins can handle tasks like code analysis, packaging, and deployment.
For example, the ‘maven-compiler-plugin’ helps in setting the Java version for compiling your code.
Integrating Maven with Other Tools
Maven plays well with other tools in the development ecosystem. Whether it’s a Continuous Integration (CI) server like Jenkins or a testing framework like JUnit, integrating Maven with various tools can lead to even more efficient project workflows.
Conclusion
Summary of Key Points
- Define Maven?: A tool for managing Java project builds.
- Why use it?: To simplify project management and dependency handling.
- Setting it up: Install, configure, and start a project quickly.
- POM understanding: The backbone of any Maven project.
- Building with Maven: Efficiently manage your project lifecycle.
The Importance of Maven in Project Management
In a world where time is of the essence, and collaboration is key, using Maven can greatly simplify project management for developers. By embracing Maven, you’re setting yourself up for better organization, clarity, and efficiency in your projects.
FAQs
- How does Maven compare to other build tools?Maven stands out due to its dependency management and standard project structure, making it easier to kickstart projects compared to tools like Ant.
- Can I use Maven for non-Java projects?While Maven is Java-centric, it can be adapted for other languages, though tools specific to those languages might be more effective. Always assess your project needs before deciding!
Happy coding with Maven! I hope this guide answer your Define Maven question and helps you embark on your project management
9 Responses